How My Site Works
What I used in the past
Previously I used Wordpress, because I have experience in it from work. It was the first platform I got acquainted to for web development. Wordpress is an excellent CMS, but I think it was an overkill for my needs. It was big and clunky, adding posts required a web based CMS, slow, insecure, need I continue? It was such a pain in the ass that I found myself not wanting to write just because of how much of a pain in the ass it was to do so. I'm a developer who loves vim and the command line.
Wordpress had to go.
Hugo to the rescue
Hugo is a static site generator. It's fast. The sites it generates are just HTML pages; no databases, no back-end API calls, and in my case, no JavaScript. On top of that, posts are just markdown documents.
example:
+++
title="How My Site Works"
date="2019-01-27"
tags=["misc"]
+++
content..
Hugo uses a decent enough templating engine that allows you to personalize the site. There are countless themes available. And you can always modify an existing theme to your liking. That's what I have done. This site takes its inspiration from the hugo-xmin theme.
What's cool about hugo content being in markdown is that it is easy to store in source code management software. I am personally partial to Git and GitHub.
Continuous Delivery and Deployment
This sounds very fancy but it's extremely simple, some would even say ghetto. The crux of this is GitHub webhooks. Whenever I make changes to the site, I commit them into my site's github repo and push the changes up into GitHub. GitHub then triggers a webhook which my web server handles. This is the web server:
const exec = require('exec');
const path = require('path');
const express = require('express')
const app = express()
var config = require('./config.js');
function build() {
exec(path.join(__dirname, 'build.sh'),
function (error, stdout, stderr) {
console.log('stdout: ' + stdout);
console.log('stderr: ' + stderr);
if (error !== null) {
console.log('exec error: ' + error);
}
});
}
app.get('/', function (req, res) {
build();
res.sendStatus(200);
});
app.post('/', function (req, res) {
build();
res.sendStatus(200);
})
app.listen(config.port, function () {
});
As you can see, it is a dead simple express app that just executes a shell script whenever it receives a post or get request.
The build script triggered is as follows:
#!/bin/bash
git pull
hugo
post.bat file used to push things to github:
git add -u
git add content/post/*.md
git add content/short/*.md
git add content/trump/*.md
git add content/improvements/*.md
git add content/journal/*.md
git add static/images/*.jpg
git add static/images/*.png
git commit -m "update"
git push -u origin master
So the script pulls the latest changes off GitHub and then rebuilds the site. The output public directory is hosted via nginx. That's it.
This key-bind executes the above script and publishes the changes:
au FileType markdown nnoremap
This allows me to make new posts, and modify existing posts by modifying files using vim. It's super convenient. And it's super fast. You can forget about performance like this from Wordpress.
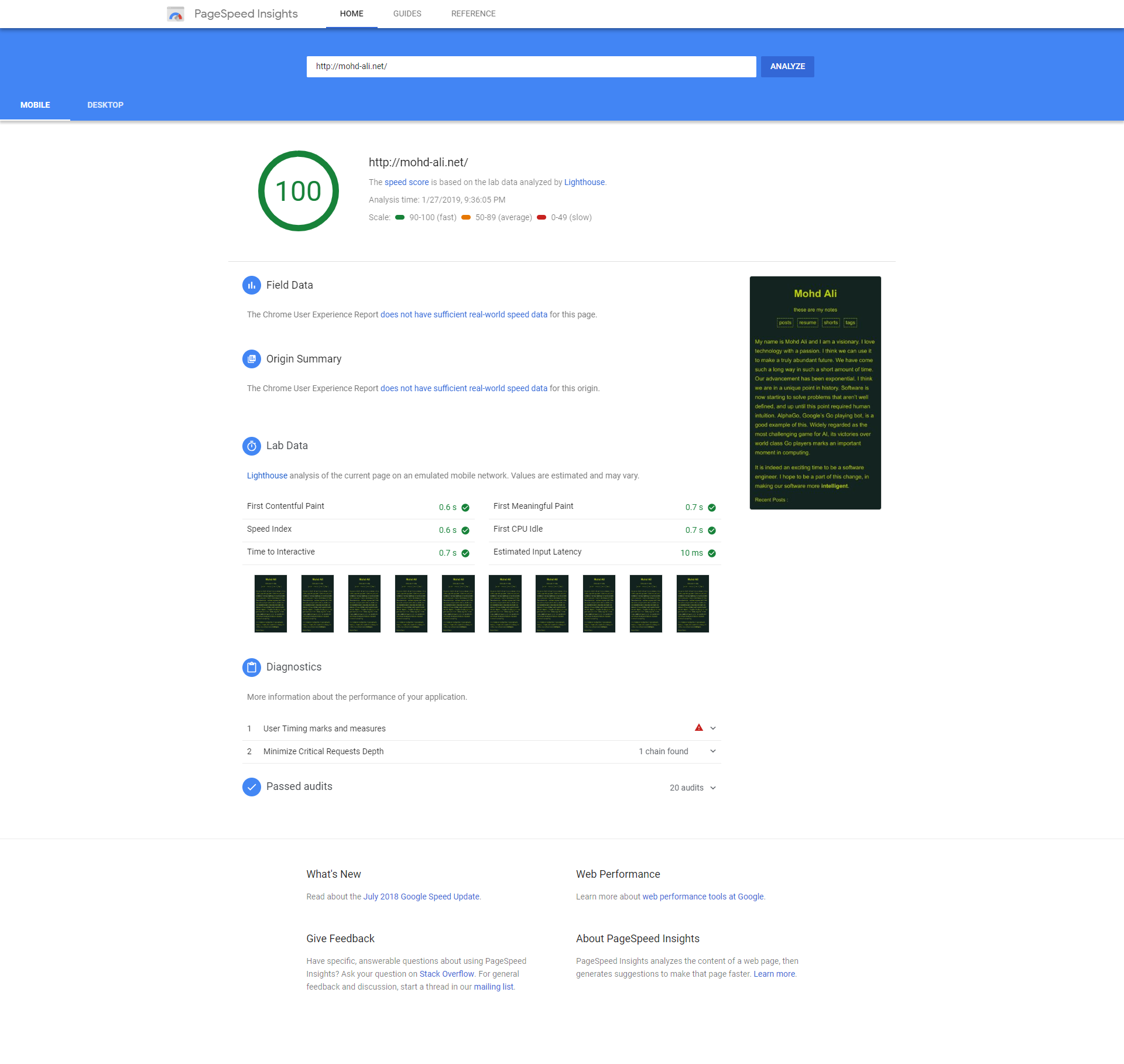
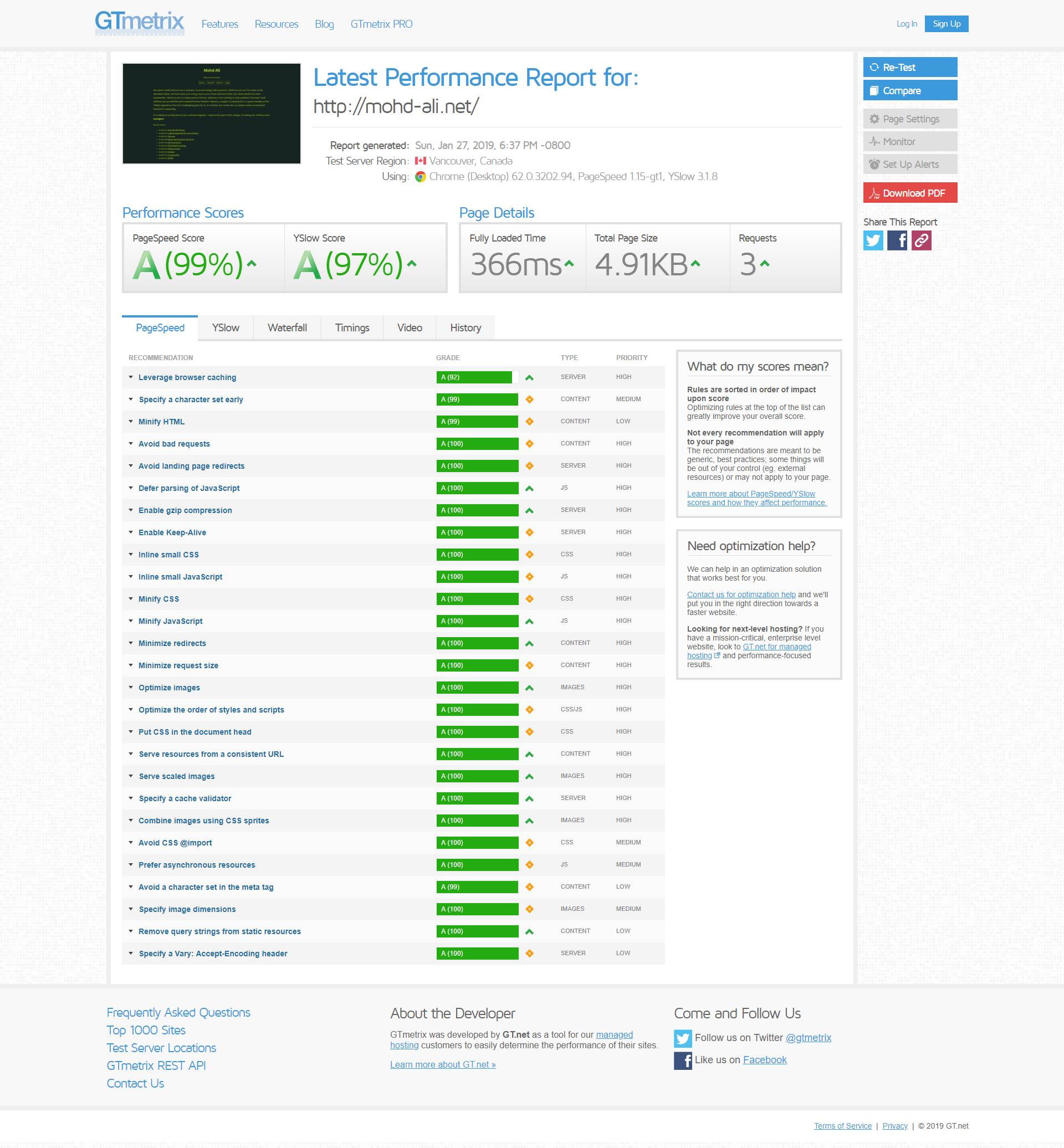
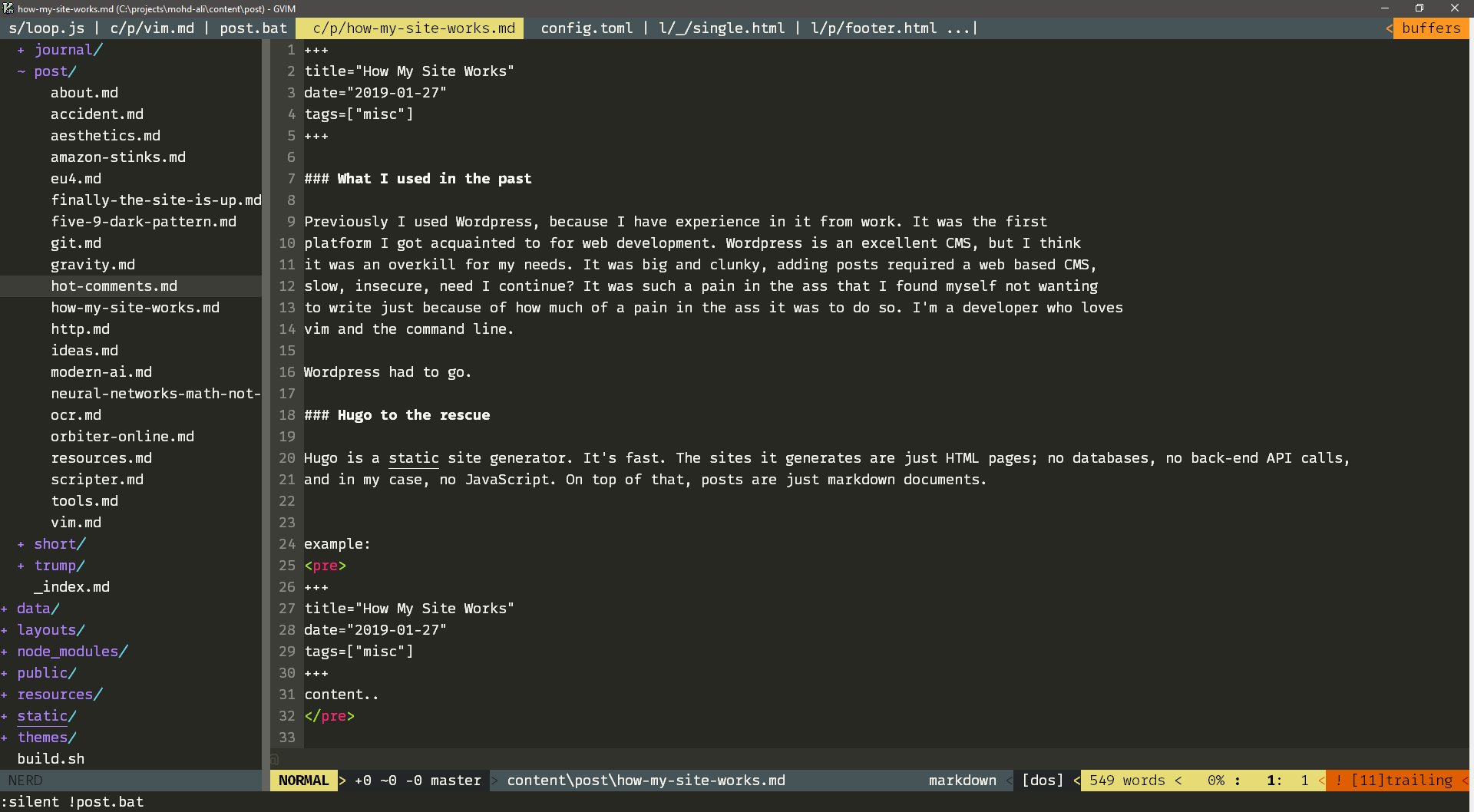